Building a Bluetooth Bridge in React Native for Android
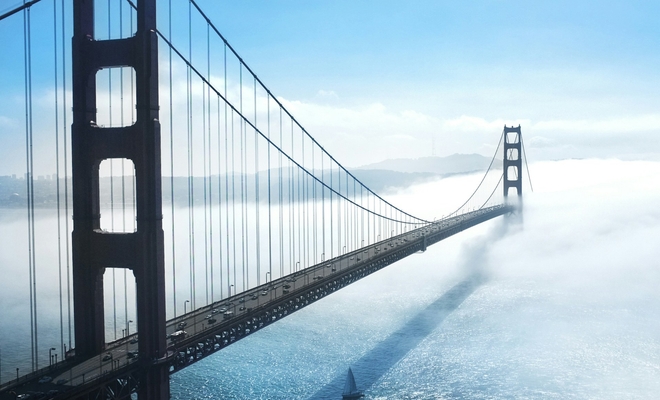
Introduction
React Native provides a powerful way to build cross-platform mobile applications, but sometimes accessing native features, like Bluetooth, requires bridging native code with JavaScript. In this guide, we'll explore how to create a custom React Native bridge to handle Bluetooth operations on Android.
Steps to Create a React Native Bridge for Bluetooth on Android
-
Set Up Your React Native Project:
- Create a new React Native project or use an existing one.
- Ensure the project has Android setup (
android
directory).
-
Create a Native Module:
- In the
android/src/main/java/com/your_project_name
directory, create a new Java class file (e.g.,BluetoothModule.java
). - Extend the
ReactContextBaseJavaModule
class. - Implement a
getName
method to return the module name.
package com.your_project_name; import com.facebook.react.bridge.ReactApplicationContext; import com.facebook.react.bridge.ReactContextBaseJavaModule; import com.facebook.react.bridge.ReactMethod; import com.facebook.react.bridge.Promise; public class BluetoothModule extends ReactContextBaseJavaModule { BluetoothModule(ReactApplicationContext context) { super(context); } @Override public String getName() { return "BluetoothModule"; } }
- In the
-
Implement Bluetooth Logic:
- Add the necessary permissions to the
AndroidManifest.xml
file.
<uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
- Use the Android
BluetoothAdapter
API within yourBluetoothModule
class. - Example: Function to check if Bluetooth is enabled.
import android.bluetooth.BluetoothAdapter; @ReactMethod public void isBluetoothEnabled(Promise promise) { BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); boolean enabled = (bluetoothAdapter != null && bluetoothAdapter.isEnabled()); promise.resolve(enabled); }
- Add the necessary permissions to the
-
Register the Module:
- Create a
Package
class to register the module. - In the
android/src/main/java/com/your_project_name
directory, create a new Java class file (e.g.,BluetoothPackage.java
). - Implement the
ReactPackage
interface.
package com.your_project_name; import com.facebook.react.ReactPackage; import com.facebook.react.bridge.NativeModule; import com.facebook.react.bridge.ReactApplicationContext; import com.facebook.react.uimanager.ViewManager; import java.util.ArrayList; import java.util.Collections; import java.util.List; public class BluetoothPackage implements ReactPackage { @Override public List<NativeModule> createNativeModules(ReactApplicationContext reactContext) { List<NativeModule> modules = new ArrayList<>(); modules.add(new BluetoothModule(reactContext)); return modules; } @Override public List<ViewManager> createViewManagers(ReactApplicationContext reactContext) { return Collections.emptyList(); } }
- Create a
-
Add the Package to MainApplication:
- Edit the
MainApplication.java
file to include the new package.
import com.your_project_name.BluetoothPackage; @Override protected List<ReactPackage> getPackages() { return Arrays.<ReactPackage>asList( new MainReactPackage(), new BluetoothPackage() // Add this line ); }
- Edit the
-
Use the Module in JavaScript:
- Import the module in your JavaScript file and call its methods.
import { NativeModules } from 'react-native'; const { BluetoothModule } = NativeModules; BluetoothModule.isBluetoothEnabled() .then((enabled) => console.log('Bluetooth enabled:', enabled)) .catch((error) => console.error(error));
Important Considerations
- Permissions: Android requires specific permissions for Bluetooth. Make sure to request runtime permissions on Android versions requiring them.
- Error Handling: Properly handle errors, especially related to Bluetooth hardware availability or permissions.
- Lifecycle Management: Ensure that Bluetooth resources are properly released when the module is no longer needed.
This guide provides a basic approach to creating a Bluetooth bridge in React Native. The implementation can vary based on the features you want to provide, but this approach gives you a starting point for integrating Bluetooth functionality into your React Native Android applications.